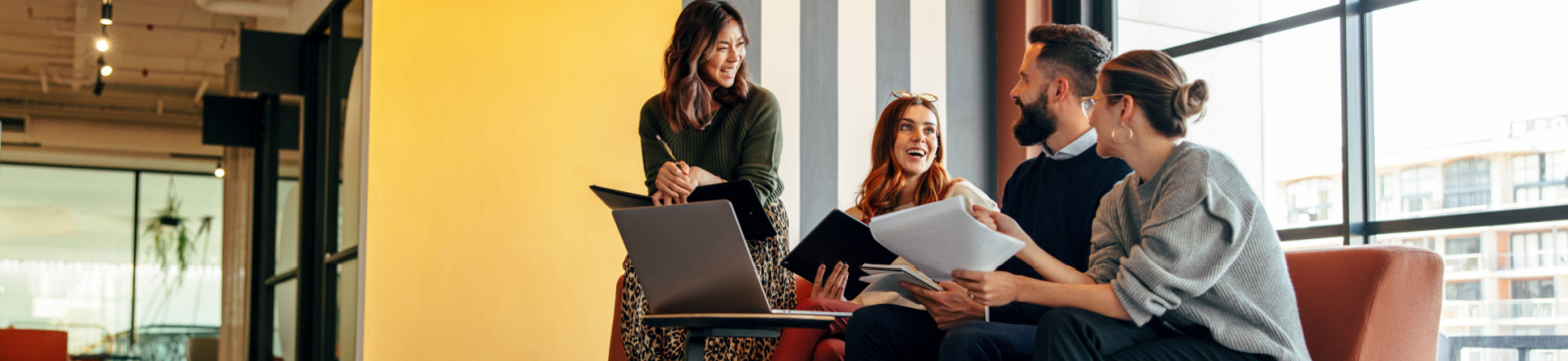
This course will change the way you look at your C# code. We'll teach you the common weaknesses and their consequences that can allow hackers to attack your system, and – more importantly – best practices you can apply to protect yourself. We give you a holistic view on the security aspects of the .NET framework – such as making use of cryptography or Code Access Security – as well as common C# programming mistakes you need to be aware of.
Interested in attending? Have a suggestion about running this event near you?
Register your interest now
Description
Module 1: IT security and secure coding
Lessons:
- Nature of security
- What is risk?
- IT security vs. secure coding
- From vulnerabilities to botnets and cybercrime
- Nature of security flaws
- Reasons of difficulty
- From an infected computer to targeted attacks
- The Seven Pernicious Kingdoms
- OWASP Top Ten 2017
Module 2: Web application security
Lessons:
- Injection
- Injection principles
- SQL injection
- Exercise – SQL Injection
- Exercise – SQL injection
- Typical SQL Injection attack methods
- Blind and time-based SQL injection
- SQL injection protection methods
- Other injection flaws
- Command injection
- Command injection exercise – starting Netcat
- Case study – ImageMagick
- Cookie injection / HTTP parameter pollution
- Exercise – Value shadowing
- Broken authentication
- Session handling threats
- Session fixation
- Exercise – Session fixation
- Session handling best practices
- Setting cookie attributes – best practices
- Sensitive data exposure
- Transport layer security
- XML external entity (XXE)
- XML Entity introduction
- XML bomb
- Exercise – XML bomb
- XML external entity attack (XXE) – resource inclusion
- XML external entity attack – URL invocation
- XML external entity attack – parameter entities
- Exercise – XXE attack
- Preventing entity-related attacks
- Case study – XXE in Google Toolbar
- Broken access control
- Typical access control weaknesses
- Insecure direct object reference (IDOR)
- Exercise – Insecure direct object reference
- Protection against IDOR
- Case study – Facebook Notes
- Failure to restrict URL access
- Security misconfiguration
- Configuration management
- Hardening
- Patch management
- ASP.NET components and environment overview
- Insecure file uploads
- Exercise – Uploading executable files
- Filtering file uploads – validation and configuration
- Cross-Site Scripting (XSS)
- Persistent XSS
- Reflected XSS
- DOM-based XSS
- Exercise – Cross Site Scripting
- Exploitation: CSS injection
- Exploitation: injecting the <base> tag
- Exercise – HTML injection with base tag
- XSS prevention
- Output encoding API in C#
- XSS protection in ASP.NET – validateRequest
- Web Protection Library (WPL)
- Insecure deserialization
- Deserialization basics
- Security challenges of deserialization
- Deserialization in .NET
- From deserialization to code execution
- POP payload targeting MulticastDelegate (C#)
- Real-world .NET examples of deserialization vulnerabilities
- Issues with deserialization – JSON
- Best practices against deserialization vulnerabilities
- Using components with known vulnerabilities
- Vulnerability attributes
- Common Vulnerability Scoring System – CVSS
Module 3: Client-side security
Lessons:
- JavaScript security
- Same Origin Policy
- Cross Origin Resource Sharing (CORS)
- Exercise – Client-side authentication
- Client-side authentication and password management
- Protecting JavaScript code
- Exercise – JavaScript obfuscation
- Clickjacking
- Exercise – Do you Like me?
- Protection against Clickjacking
- Anti frame-busting – dismissing protection scripts
- Protection against busting frame busting
- AJAX security
- XSS in AJAX
- Script injection attack in AJAX
- Exercise – XSS in AJAX
- XSS protection in Ajax
- Exercise CSRF in AJAX – JavaScript hijacking
- CSRF protection in AJAX
- HTML5 security
- New XSS possibilities in HTML5
- HTML5 clickjacking attack – text field injection
- HTML5 clickjacking – content extraction
- Form tampering
- Exercise – Form tampering
- Cross-origin requests
- HTML proxy with cross-origin request
- Exercise – Client side include
Module 4: .NET security architecture and services
Lessons:
- .NET architecture
- Code Access Security
- Full and partial trust
- Evidence classes
- Permissions
- Code access permission classes
- Deriving permissions from evidence
- Defining custom permissions
- .NET runtime permission checking
- The Stack Walk
- Effects of Assert()
- Class and method-level declarative permission
- Imperative (programmatic) permission checking
- Exercise – sandboxing .NET code
- Using transparency attributes
- Allow partially trusted callers
- Exercise – using transparency attributes
Module 5: Practical cryptography
Lessons:
- Cryptosystems
- Elements of a cryptosystem
- Symmetric-key cryptography
- Providing confidentiality with symmetric cryptography
- Symmetric encryption algorithms
- Block ciphers – modes of operation
- Other cryptographic algorithms
- Hash or message digest
- Hash algorithms
- SHAttered
- Message Authentication Code (MAC)
- Providing integrity and authenticity with a symmetric key
- Random numbers and cryptography
- Cryptographically-strong PRNGs
- Hardware-based TRNGs
- Asymmetric (public-key) cryptography
- Providing confidentiality with public-key encryption
- Rule of thumb – possession of private key
- Combining symmetric and asymmetric algorithms
- Public Key Infrastructure (PKI)
- Man-in-the-Middle (MitM) attack
- Digital certificates against MitM attack
- Certificate Authorities in Public Key Infrastructure
- X.509 digital certificate
Module 6: Common coding errors and vulnerabilities
Lessons:
- Input validation
- Input validation concepts
- Integer problems
- Representation of negative integers
- Integer overflow
- Exercise IntOverflow
- What is the value of Math.Abs(int.MinValue)?
- Integer problem – best practices
- Case study – Integer overflow in .NET
- Path traversal vulnerability
- Path traversal mitigation
- Case study – Insufficient URL validation in LastPass
- Unvalidated redirects and forwards
- Unsafe native calls
- Exercise – Unsafe unmanaged code
- Unsafe reflection
- Implementation of a command dispatcher
- Unsafe reflection – spot the bug!
- Mitigation of unsafe reflection
- Log forging
- Some other typical problems with log files
- Improper use of security features
- Typical problems related to the use of security features
- Insecure randomness
- Weak PRNGs in .NET
- Password management
- Exercise – Weakness of hashed passwords
- Password management and storage
- Special purpose hash algorithms for password storage
- Argon2 and PBKDF2 implementations in .NET
- bcrypt and scrypt implementations in .NET
- Case study – the Ashley Madison data breach
- Typical mistakes in password management
- Exercise – Hard coded passwords
- Accessibility modifiers
- Accessing private fields with reflection in .NET
- Exercise Reflection – Accessing private fields with reflection
- Improper error and exception handling
- Typical problems with error and exception handling
- Empty catch block
- Overly broad catch
- Using multi-catch
- Catching NullReferenceException
- Exception handling – spot the bug!
- Exercise – Error handling
- Exercise – Information leakage through error reporting
- Time and state problems
- Concurrency and threading
- Concurrency in .NET
- Omitted synchronization – spot the bug!
- Exercise – Omitted synchronization
- Incorrect granularity – spot the bug!
- Exercise – Incorrect granularity
- Deadlocks
- Avoiding deadlocks
- Exercise – Avoiding deadlocks
- Lock statement
- Serialization errors (TOCTTOU)
- TOCTTOU example
- Exercise – Race condition
- Exercise – Exploiting the race condition
- Code quality problems
- Dangers arising from poor code quality
- Poor code quality – spot the bug!
- Unreleased resources
- Serialization – spot the bug!
- Exercise – Serializable sensitive
- Private arrays – spot the bug!
- Private arrays – typed field returned from a public method
- Class not sealed – object hijacking
- Exercise – Object hijacking
- Immutable string – spot the bug!
- Exercise – Immutable strings
- Using SecureString
Module 7: Principles of security and secure coding
Lessons:
- Matt Bishop’s principles of robust programming
- The security principles of Saltzer and Schroeder
Module 8: Knowledge sources
Lessons:
- Secure coding sources – a starter kit
- Vulnerability databases
- .NET secure coding guidelines at MSDN
- .NET secure coding cheat sheets
- Recommended books – .NET and ASP.NET
Audience Profile
C# Web developers
Prerequisites
General C# and Web application development skills are required.